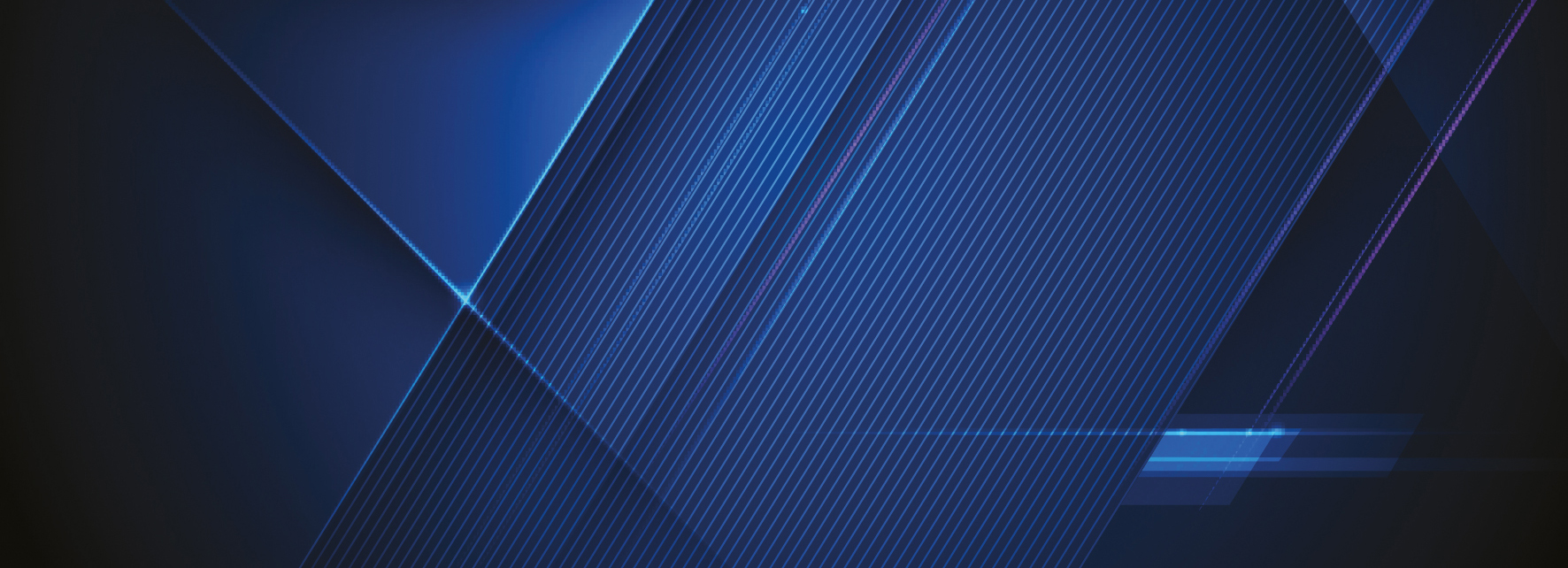
Sending and receiving SMS through HTTP
Overview
This document describes the HTTP interface between a content provider and YoYo Mobile. This HTTP interface is used to send and receive SMS messages to end users through YoYo's mobile platform.
Sending messages
To send an SMS message to an enduser the content provider makes an HTTP request to YoYo's HTTP server. This HTTP request contains several parameters that indicates who the message is for, what type of message it is, the message content and more.
The URL that should be used by the content provider to send HTTP requests will be given to the content provider when an agreement has been signed. The content provider also needs to give YoYo a list of the IP adresses that the user will send requests from, so that we can open up our rewall.
For SMS messages, parameters can be sent with either GET method and url parameters or POST method with a content type of application/x-www-form-urlencoded.
Charset
The content provider will be given two urls for sending SMS messages. One will use the ISO-8859-1 characterset, and the other will use UTF-8. It's up to the contentprovider to choose which to use.
Note that all characters outside of the US-ASCII 7-bit subset must be urlencoded.
Also note that all characters for normal SMS messages must be within the GSM characterset. Sending msg parameter with characters outside the GSM characterset will result in an error.
Parameters
The content provider must set the following parameters when sending an HTTP request. All parameters are to be handled as strings.
username The username for the service. Given by YoYo.
password The password for the service. Given by YoYo.
msn The msn or mobile subscriber number. This is the mobile number that this message will be delivered to. This number should always include the country code, but not the + sign.
type The type of message that is to be sent. Described further in the section
below.
prex The prex that the message will be registered on. A list of allowed prexes will be specied together with the content provider in the agreement. This is used for statistics registration.
price The price the end user will pay for this message. A list of allowed prices is specic for the country and access number in use. A list of allowed prices will be given to the content provider in each case. For bulk messages this parameter can be omitted.
accessnr The access number that this message will be sent out on. YoYo's main number is 2010. For bulk messages, accessnr should be set to bulk
inmsgid The id of the message that triggered this message. When receiving messages from YoYo with HTTP, set this inmsgid parameter to the inmsgid parameter that you got from the receiving message. This parameter is not required, but should be set when available.
originator The originator of the message. This parameter is only valid if accessnr is set to bulk, for other messages originator depends on the accessnr used. The originator can consist of up to 11 characters from the regular SMS character set.
Content types
The type parameter describes what kind of content the message contains.
Allowed values for the type parameter are
text Plain text with a maximum length of 160 characters.
longtext Same as plain text, however length can exceed 160 characters.
Text messages
When type is set to text a simple SMS message is sent. Type text can only contain up to 160 characters, if you try to send more an error will be returned.
When type is set to longtext the text message can be more than 160 characters. Message will be split in to more than one SMS if necessary.
All modern mobile phones are able to transparently concatenate the long messages.
The following extra parameters must be set for text messages:
msg The message text.
Responses
The content provider should always check that the result is ok. If a message can not be sent it is essential to check the error message before reporting an error to YoYo.
Success response
If all goes well and the message can be sent the response will be
Status:OK
Description:1234
where the description will contain the message id. The message id is a unique identier of the message in the YoYo database.
Error response
If something goes wrong the response will be
Status:Error
Description:An error message
where the description will indicate what went wrong.
Status reports
YoYo can send out status reports for each message when we receive the status from the operator. If this is wanted an URL must be registered in YoYo's database for status report. This url will be requested, through an HTTP GET method, each time a status report is ready. The following parameters will be set:
msgid The messageid as returned when sending a message.
status The statuscode of the message.
Status codes
The following statuscodes can be set:
10 The message was billed ok.
11 The message was sent ok, but message is not billable.
20 The message failed with unknown reason.
21 The message could not be delivered to the end user.
22 The end-user has no balance on pre-paid account.
23 The end-user has been barred by the operator.
24 The end-user is no longer a customer of the operator.
25 The end-user has requested barring of overcharged messages.
26 The end-user is not old enough for this service
27 The end-user has exceeded purchase limit
Receiving messages
The content provider must supply a URL to YoYo which will be used to forward SMS messages received from end users.
When YoYo receives an SMS message which is to be delivered to the content provider, YoYo will issue an HTTP request to the content provider's HTTP server.
Parameters will be sent to the content provider with the HTTP request. For sms messages the parameters can be sent using GET method with url parameters or POST method with content-type set to application/x-www-form-urlencoded.
Parameters
type The type of message.
prex The prex that this message was received on.
accessnr The access number that this message was received on. YoYo's main number is 2010.
inmsgid The id of the message. This id identies this message in YoYo's database. It should be used when sending message replies.
operator The name of the operator.
msg The text message.
Responses
The content provider should send a message back to YoYo with the following
content:
Message received ok
It's important that the content provider sends this reply, so that YoYo can be sure that the message has been delivered correctly.
Examples
Example of sending text sms message
https://someurl/somefile?username=cpuser&password=cppassword&msn=4741560905&type=text&msg=This+is+a+test+message&prefix=TEST&price=NOK+10.00&accessnr=2010
This will send a text message to 4741560905 with the content «This is a test message». The message will be registered on the TEST prex on accessnr 2010. The message will cost NOK 10.00 for the end user. The username is set to cpuser and the password to cppassword. This example uses the GET method to send the parameters.
Example of receiving text message
https://someurl/somefile?msn=4741560905&type=text&msg=This+is+a+test+message&prefix=TEST&accessnr=2010
Example of sending and receiving in PHP
This example show a basic «echo» service written in PHP. It will receive SMS and reply with «You wrote: » and the text you wrote.
<?php
$username = "testuser";
$password = "testpassword";
$accessnr = "2010";
$country = "norway";
$prefix = "TEST";
$price = "NOK 1.00";
$type = "text";
$msn = $_REQUEST['msn'];
$inmsgid = $_REQUEST['inmsgid'];
$msg = "You wrote: " . $_REQUEST['msg'];
$url = "https://smsgw.yoyo.no/api/compatrequest?";
$url .= "username=" . urlencode($username);
$url .= "&password=" . urlencode($password);
$url .= "&accessnr=". urlencode($accessnr);
$url .= "&country=" . urlencode($country);
$url .= "&prefix=" . urlencode($prefix);
$url .= "&price=" . urlencode($price);
$url .= "&type=" . urlencode($type);
$url .= "&msn=" . urlencode($msn);
$url .= "&inmsgid=" . urlencode($inmsgid);
$url .= "&msg=" . urlencode($msg);
$file_array = @file($url);
if ($file_array == false) {
error_log("Communications agains SMS server failed");
} else if ($file_array[0] == "Status:OK\n") {
// Everything ok
} else {
error_log("SMS error: " . $file_array[1]);
}
echo "Message received ok";
?>